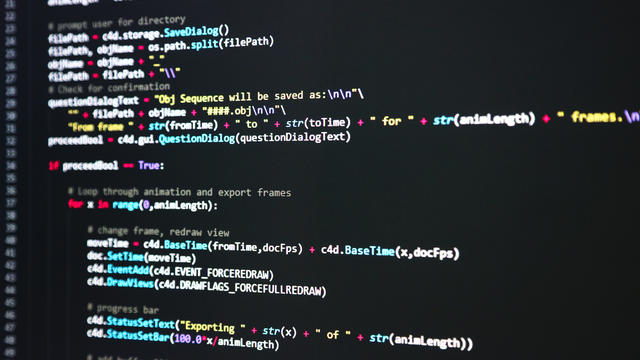
Now that you have seen how to structure data in lists and dictionaries, it is time to add some structure to your programs. In this lesson you will start with the Python development environment Thonny and write your first Python programs.
Until now you already have your Pythoncommands are executed in an interactive session in the Python interpreter. This is useful for some small calculations, but for more complex matters you need to write a complete program. And that’s what we’re going to do in this lesson, where we also see many commands that Python offers to build a program.
Thonny
If you are starting to write Python programs that do more than simple operations, the Python interpreter will not suffice: you will need a development environment that will help you build your program. In this lesson we use Thonny, which is installed by default with Python from version 3.7.
When you start up Thonny, you will see two text fields. Enter your Python program in the text field at the top. The text field at the bottom is a ‘shell’: this is the same as the Python interpreter we used in the previous lessons. This text field can be useful for trying out some commands while you are writing a program.
True or False
But before we write a program, we need to explain one more Python datatype, a very simple one: bool. This represents a boolean value: true or false, or in Python: True or False. Many functions and operators in Python return one of these values. Just enter the following commands into Thonny’s shell text field:
>>> names = [‘lies’, ‘jan’, ‘kees’, ‘mireille’, ‘koen’, ‘rob’]
>>> ‘kees’ in names
True
>>> ‘aniek’ in names
False
>>> names[0] == ‘groin’
True
>>> names[1] ! = ‘kees’
True
>>> 2 <5
True
>>> 2> 5
False
>>> 3.1.is_integer ()
False
>>> 3.0.is_integer ()
True
As you can see, you can use in test whether an element is in a list. With == you test for the equality of two objects and with != on inequality. Equations like < (less than) and > (greater than) also return a boolean value. In addition, you also have <= (less than or equal to) and >= (greater than or equal to). And a function such as is_integer () is useful for determining whether a float is an integer value.
You can also ‘calculate’ with Boolean values ​​themselves, namely with the operators and, or and not. The expression X or y is True if x or y or both objects are True; x and y is True if both objects True to be; and not x is True as x False is and vice versa.
Test values: if
Then we are now going to write our first Python program. The program asks the user to enter an integer from 1 to 10 and then displays the number written in a word. But if the user enters a number less than 1 or greater than 10, he will get an error. The program looks like this:
numbers = {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: ‘four’, 5: ‘five’, 6: ‘six’, 7: ‘seven’, 8: ‘eight’ , 9: ‘nine’, 10: ‘ten’}
number = int (input (“Enter an integer from 1 to 10:”))
if number <1:
print (“The number is less than 1.”)
elif number> 10:
print (“The number is greater than 10.”)
else:
print (numbers[getal])
On the first line we create a dictionary with the numbers from 1 to 10 as key, with a string with the number expressed in a word as the corresponding value. Then we call the function input with as argument a string asking to enter a number. The function input returns as value the string entered by the user. We put that string with int to switch to an integer, provided of course that the user actually entered a number.
Then we test with if number <1 or the number is less than 1. In that case the line after it is executed. If that first condition is not met, Python checks the condition in the rule elif number> 10. If so, the rule will be executed after it; if not, the line will be after else: executed.
Such a block as above can be used for multiple checks elif contain: these are then tested one by one until a condition is met. Incidentally, the controls are met elif and else optional. So you can easily only with if test a condition: in case that condition is not met, nothing happens and the program continues as usual.
Run your program
Enter the code in the program text field of Thonny. You notice that Thonny automatically indents the lines with four characters after you press Enter after a line with if, elif or else. Note: without this indent, the Python code is not valid. After typing in the complete code, save the file in Thonny. Then press the green icon with the triangle to run the program.
If you made a typo, you will see an error message in the bottom text field. This is the case, for example, if you enter a: at the end of a line with if, elif or else forget or if you don’t indent the code correctly. Then correct your code and run the program again.
Your program will now ask you to enter a number from 1 to 10 in the text field at the bottom. Try out the different parts of your program by entering a number that is too small, too large or within range. After each entry, press Enter and you will see the result. Then run the program again for another attempt.
Run programs on the command line
You don’t have to run your programs in Thonny. If you go to the directory where you saved your Python program on Command Prompt (on Windows) or Terminal (on Linux or macOS), you can run the program with the command python3 name of program.py.
A range of numbers
Our program is more complicated than necessary. We don’t really need to know if the number entered is less than 1 or greater than 10: we just want to know if it is in the requested range of 1 to 10. We can use the function for this range, which represents a range of numbers. In this way we can simplify our previous program to:
numbers = {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: ‘four’, 5: ‘five’, 6: ‘six’, 7: ‘seven’, 8: ‘eight’ , 9: ‘nine’, 10: ‘ten’}
number = int (input (“Enter an integer from 1 to 10:”))
if number – 1 in range (len (numbers)):
print (numbers[getal])
else:
print (“The number is not in the range 1 to 10.”)
We now only have one test with if necessary, without elif. After all, in that check we test whether the entered number is within the range. We calculate this range by passing the length of the dictionary with numbers to the function range. Since Python always starts counting from 0, the range for that dictionary with length 10 equals the numbers from 0 to 9. But since we start counting humans from 1, we subtract 1 from the entered number before testing whether number is in range. And this is how we arrive at this line:
if number – 1 in range (len (numbers)):
Conditional loops
Our program has one more major flaw: if the user enters a value out of range, the program simply quits and the user has to run the program again. In that case, why can’t the program ask for a number again by itself? That is possible, namely with while:
numbers = {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: ‘four’, 5: ‘five’, 6: ‘six’, 7: ‘seven’, 8: ‘eight’ , 9: ‘nine’, 10: ‘ten’}
number = 0
while number – 1 not in range (len (numbers)):
number = int (input (“Enter an integer from 1 to 10:”))
print (numbers[getal])
As a rule with while we test with not in or the entered number not within range. In that case, the indented command below it will be executed. And then the test is usually done with while again, as long as the number is not in range. Only when the number is within range does the while block stop and the program continues with the last line. We call such a while block a loop, because the program runs in circles, as it were, as long as the condition on the while line is satisfied.
Note: we know at the beginning of the program 0 to the variable number so that the while loop is executed the first time anyway.
Resume
In this lesson, we’ve moved from individual Python commands to Python programs. We did this in the Thonny development environment. We looked at the data type bool and worked with conditions ifblocks and whileloops. We also used the range function for a range of numbers. We used input and print for input and output. The next lesson will be devoted entirely to input and output, as well as how to move through elements in a list or dictionary.
Order
Our latest program has one more drawback: when entering a number outside the range from 1 to 10, it simply asks for another number without any additional notification that you have typed something wrong. Add that extra notification, as we do in the program with if had.
Elaboration
numbers = {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: ‘four’, 5: ‘five’, 6: ‘six’, 7: ‘seven’, 8: ‘eight’ , 9: ‘nine’, 10: ‘ten’}
number = int (input (“Enter an integer from 1 to 10:”))
while number – 1 not in range (len (numbers)):
print (“The number is not in the range 1 to 10.”)
number = int (input (“Enter an integer from 1 to 10:”))
print (numbers[getal])
This program combines the ideas behind our two previous programs. We initialize number now not with 0, but with user input. This first time, if the number is immediately in the range 1 to 10, the while loop condition is not met and the number is displayed. In the other case, the loop is started, whereby as long as the number is not in range, the user is notified and prompted to enter a number.
Cheat sheet
argument: a value that you pass to a function.
bool (‘boolean value’): true (True) or false (False).
if: a test whether a condition has been met.
development environment (development environment): software that supports you in writing programs.
while: a loop that is executed as long as a condition is met.
.