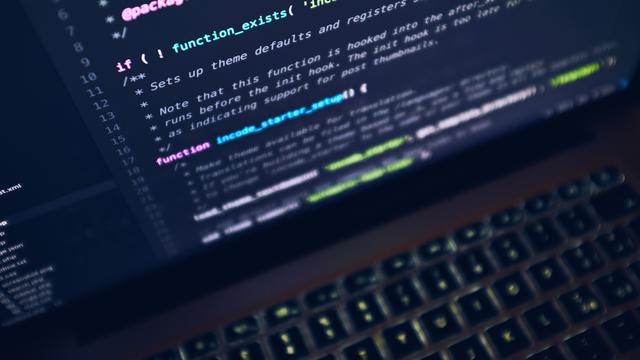
You can already handle all kinds of aspects of Python well, but in addition to the standard Python library, there are many extra modules. In this lesson you will learn how to install these and how to work with virtual environments to deal with conflicting module requirements.
Basic functionality that almost everyone needs is in the standard library of Python. In lesson 6 you already saw how to access this functionality with the assignment import and we briefly showed you some standard modules. But in that lesson you also wrote your own module. This lesson is all about installing additional modules that others have written.
Find packages on PyPI
Anyone can create their own Python module, as you did in lesson 6. Python offers a central repository with modules that you can easily install and use in your own Python programs: the Python Package Index or PyPI for short. You can find them on the website www.pypi.org.
PyPI contains more than 170,000 projects called packages. A package contains one or more Python modules. You search the PyPI website for keywords to find packages to use in your code. But it can be even easier on the command line of your operating system with the program pip3, which is part of Python. Here’s how to search for packages with the following command:
pip3 search
You will then see a list of package names and a short description of one line containing your search term. If you want to read more information about the package, you will need to refer to the package page on the PyPI website, which has a more detailed description, including a link to the package website.
Install a package with pip3
Once you have found a package that you want to use, you can also easily install it with pip3:
pip3 install urllib3
After installing a package, you can request more information about the package with:
pip3 show urllib3
In addition to the short description and version number of the installed package, you will also see the website and the license.
Using installed module in your Python code
After installing a package with pip3, you can use the modules in it, just like the standard Python modules. For the example of urllib3:
>>> import urllib3
>>> http = urllib3.PoolManager ()
>>> r = http.request (‘GET’, ‘http://reshift.nl/robots.txt’)
>>> r.status
200
>>> r.data
b ‘User agent: * nDisallow: / wp-admin / nAllow: /wp-admin/admin-ajax.phpn’
After the installation of a package, it no longer matters whether the module is in the standard library or not. Before using a module in your own code, you should check what the license of the project allows you to do. However, since most Python packages use an open source license, use will not be problematic anytime soon.
The usefulness of virtual environments
If you install a lot of Python packages with pip3, you might run into problems. After all, packages also import other packages that use them, which we call ‘dependencies’. But maybe Package A will install version 1.0 of package Z and package B will install the version 1.0 incompatible version 2.0 of package Z. The result? After installing package B, package A no longer works, because you can only install one version of a Python package.
Fortunately, Python has a solution for these problems: virtual environments. A virtual environment is a directory on your computer where you install Python packages instead of your global Python package directory. This virtual environment is completely separate from other virtual environments and from the global Python package directory. If you then create a new virtual environment for each Python project you want to install or develop, you can be sure that installing the packages you need will not cause any of your other projects to fail.
Setting up a virtual environment with venv
You can set up a virtual environment in Python with the module venv:
python3 -m venv C: path to my environment
This creates the specified directory (and any parent directories that do not already exist) and references the Python interpreter and an empty directory for packages.
To use the virtual environment now, you must first ‘activate’ it. You do that as follows:
C: path to my environment Scripts activate.bat
Now if you install packages with pip3, they will be installed in the virtual environment and not in your global Python package library. You leave the virtual environment with the assignment deactivate. You then work in your global Python environment again. You can now set up another virtual environment or activate a virtual environment that you have already created. This way you can easily switch between different projects without the installation of Python packages in one environment having an impact on another environment.
Virtual environment in macOS and Linux
The Python module venv works slightly differently in other operating systems. The directory Scripts is called in macOS and Linux bin, and Lib (with capital L) is called lib (all lowercase). Moreover, you do not activate the virtual environment with a batch file as in Windows, but with a shell script, which does not have an extension.bat has, and you activate that with the command source. You can activate the virtual environment of an environment under macOS and Linux with the command:
source mining environment / bin / activate.
Save and reinstall dependencies
If your project uses a lot of dependencies and you have them all installed in a virtual environment with pip3, you naturally want someone else running your code to be able to install all those packages as easily as possible. Fortunately, you can easily create a list of all the packages installed in the virtual environment and their versions:
pip3 freeze> requirements.txt
The file requirements.txt contains a line for each installed package in the virtual environment:
package name == version number
Then the other user can create a virtual environment on his computer and copy your Python code into it. That code will not work yet because the dependencies are not installed yet. But if you get the file requirements.txt with your code, the user only needs to run one command in the virtual environment to install all dependencies:
pip3 install -r requirements.txt
After that you can be sure that your code works on the other computers as well, at least if your Python versions are the same as well. After all, the dependencies are the same and of the same versions.
If you don’t want to record the version numbers of your dependencies, you can put them in the file requirements.txt omit, along with the == designation. You then only state the package names. This command will then install the latest version of the specified packages:
pip3 install -r requirements.txt
Resume
In this lesson, you learned how to look up additional packages in the Python Package Index and how to install them with pip3. You also learned to work with virtual environments and save the dependencies of your projects, so that you can easily install them again later in a new virtual environment. This brings us almost to the end of our beginner lesson series. In the last lesson, we’re going to develop a full Python script that you can run on the command line.
Order
Install the module arrow in your global Python environment and check if you can import them. Then create a new virtual environment and verify the module arrow is not available there. Then install as well arrow in your virtual environment, so that you can import the module there.
Elaboration
pip3 install arrow
python3 -c ‘import arrow’
After installing arrow with pip3 you can run the Python interpreter and there import arrow type, but with the option -c you can also pass Python commands directly as arguments to the Python interpreter, which is useful here to quickly check if we have the module arrow can import.
Then create a new virtual environment and activate it:
python3 -m venv C: path to my environment
C: path to my environment Scripts activate.bat
Now if we want to import the arrow module with this command:
python3 -c ‘import arrow’
Then we get an error:
Traceback (most recent call last):
File “
ModuleNotFoundError: No module named ‘arrow’
Although we have installed the module in the global Python environment, it is not available in the virtual environment as it is completely separate.
Now install the module in the virtual environment:
pip3 install arrow
After that we can import the module. The Python code in your virtual environment can now use arrow, a handy Python library for working with dates and times:
python3 -c ‘import arrow; print (arrow.utcnow (). shift (hours = -2) .humanize ()) ‘
2 hours ago
Cheat sheet
Dependencies: the packages that a piece of Python software needs for its operation.
Library: a collection of code intended for use by other programs.
package: a collection of one or more Python modules.
Repository: an online repository of packages.
Software License: a license to use a computer program, often under specific conditions.
Standard library: all modules available with a standard Python installation.
Virtual environment: a directory with a separate installation of Python and Python modules.
.